IntelliBot_v1 Library
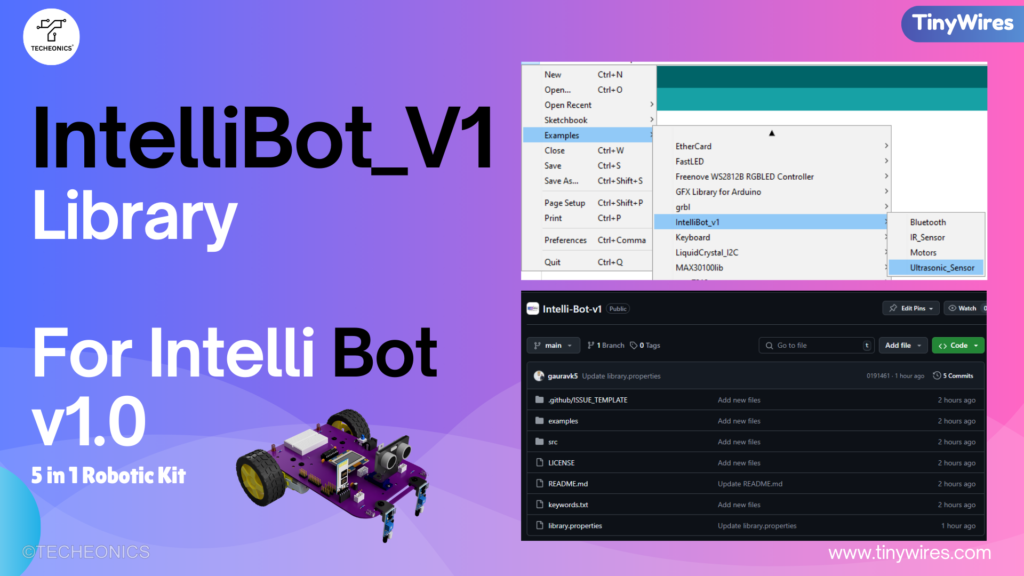
Introduction
The IntelliBot_v1 library is a powerful and user-friendly tool specifically designed for the Intelli Bot V1 DIY Robotics Kit. Whether you’re a beginner or an advanced user, this library simplifies the process of coding for robotics by providing predefined pin configurations, built-in motor control, and sensor functions. In this blog, we’ll explore the key functionalities of the IntelliBot_v1 library and how it can help you bring your robotics projects to life more easily and efficiently.
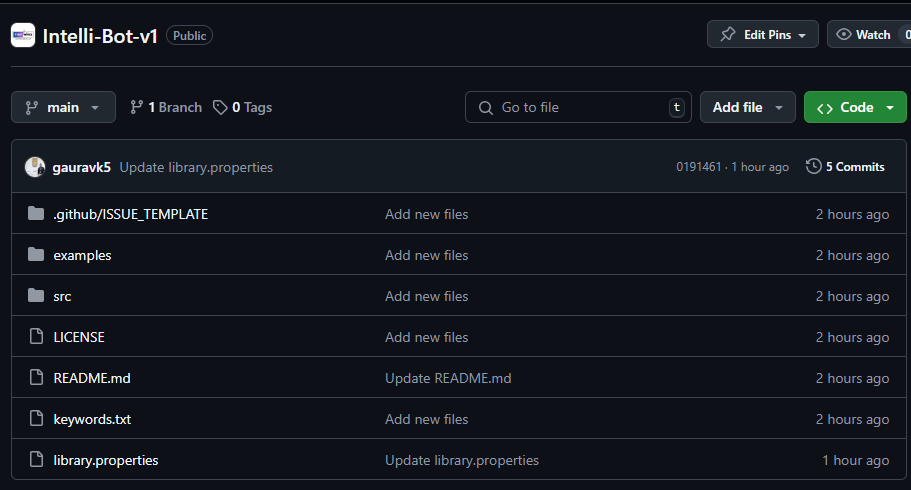
What is the IntelliBot_v1 Library?
The IntelliBot_v1 library is a custom library that eliminates the need for manually defining pins, writing complex control loops, or dealing with sensor calibration. Everything is predefined and built to work specifically with the Intelli Bot kit. The library allows you to easily control:
- Motors for movement.
- IR sensors for line following.
- Ultrasonic sensors for obstacle detection and avoidance.
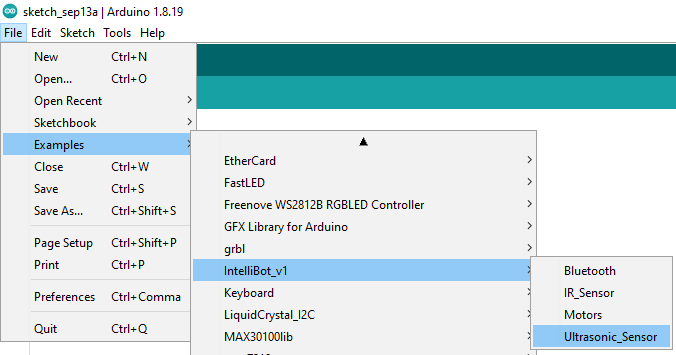
This library essentially abstracts the complexity of low-level hardware control, letting you focus on building your project.
Key Features of the IntelliBot_v1 Library
1. Predefined Pin Configuration
One of the major benefits of using this library is that all pin assignments are predefined, matching the hardware layout of the Intelli Bot kit. This means you don’t have to worry about setting up pins for motors or sensors, which drastically reduces setup time and potential errors.
Here’s how motor pin assignments look in the library:
#define MOTOR_PIN1 5
#define MOTOR_PIN2 6
These pins are already mapped for the Intelli Bot kit, so you don’t need to manually set them in your sketch.
2. Simplified Motor Control
The library provides built-in functions for motor control, simplifying robot movement. You can easily make your robot move forward, backward, or turn by calling these intuitive functions:
- Move Forward:
myMotor.forward()
- Move Backward:
myMotor.backward()
- Turn Left:
myMotor.fastLeft()
- Turn Right:
myMotor.fastRight()
- Stop:
myMotor.stop()
These functions allow you to move your robot without worrying about PWM signals or low-level motor control logic. For example, to make the robot turn right, you simply write:
myMotor.fastRight();
Example Code
Here’s a simple example of controlling the motors:
#include <IntelliBot_v1.h>
Motors myMotors;
void setup() {
myMotors.init();
}
void loop() {
myMotors.forward();
delay(2000);
myMotors.brake();
delay(1000);
myMotors.fastLeft();
delay(1000);
}
In this example:
- The robot moves forward for 2 seconds.
- It stops for 1 second.
- Then, it makes a fast left turn for 1 second.
3. IR Sensor Integration for Line Following
The IntelliBot_v1 library is designed with functionality for building a line-following robot. The library simplifies how you handle IR sensors by predefining the states for black and white colors:
const byte whiteColor = LOW;
const byte blackColor = HIGH;
With these settings, you can easily write code that makes your robot follow a black line on a white surface by checking the IR sensor states and making corresponding turns.
4. Ultrasonic Sensor Functions for Obstacle Avoidance
Creating an obstacle-avoiding robot is another essential functionality included in the IntelliBot_v1 library. The library provides functions that allow you to measure distances using an ultrasonic sensor and make decisions based on that data. This simplifies the process of integrating sensor data into your robot’s control logic, allowing for more efficient coding.
int getDistanceCm(); // get distance Value in cm
int getDistanceInch(); // get distance Value in Inch
How to Use IntelliBot_v1 Library in Your Projects
Once you have included the library in your sketch, using it is straightforward. Here’s a quick example of how to use the motor and IR sensor functionalities in a simple sketch:
#include <IntelliBot_v1.h>
void setup() {
myMotor.init();
pinMode(IR_PIN, INPUT);
}
void loop() {
if (digitalRead(IR_PIN) == whiteColor) {
myMotor.forward();
} else {
myMotor.stop();
}
}
In this example, the robot moves forward when the IR sensor detects white, and it stops when it detects black. This is a basic implementation of a line-following robot using the IntelliBot library.
Why Use the IntelliBot Library?
- Simplified Coding: The IntelliBot library allows even beginners to control motors and sensors with just a few lines of code.
- Predefined Pin Configurations: No need to manually define or guess the pin setup.
- Custom Functions: Built-in functions like motor control and sensor integration reduce complexity and coding time.
- Faster Prototyping: Pre-written examples help you prototype and develop robotic systems quickly.
- Customization: Although the library simplifies coding, it still allows you to modify or extend the functions as needed.
Conclusion
The IntelliBot_v1 library offers a simplified approach to robotics by providing pre-configured pins, motor control functions, and sensor integration. This library significantly reduces the complexity of programming robots, making it ideal for both beginners and advanced users. Whether you’re building an obstacle-avoiding bot or a line-follower